Button Reading Guide
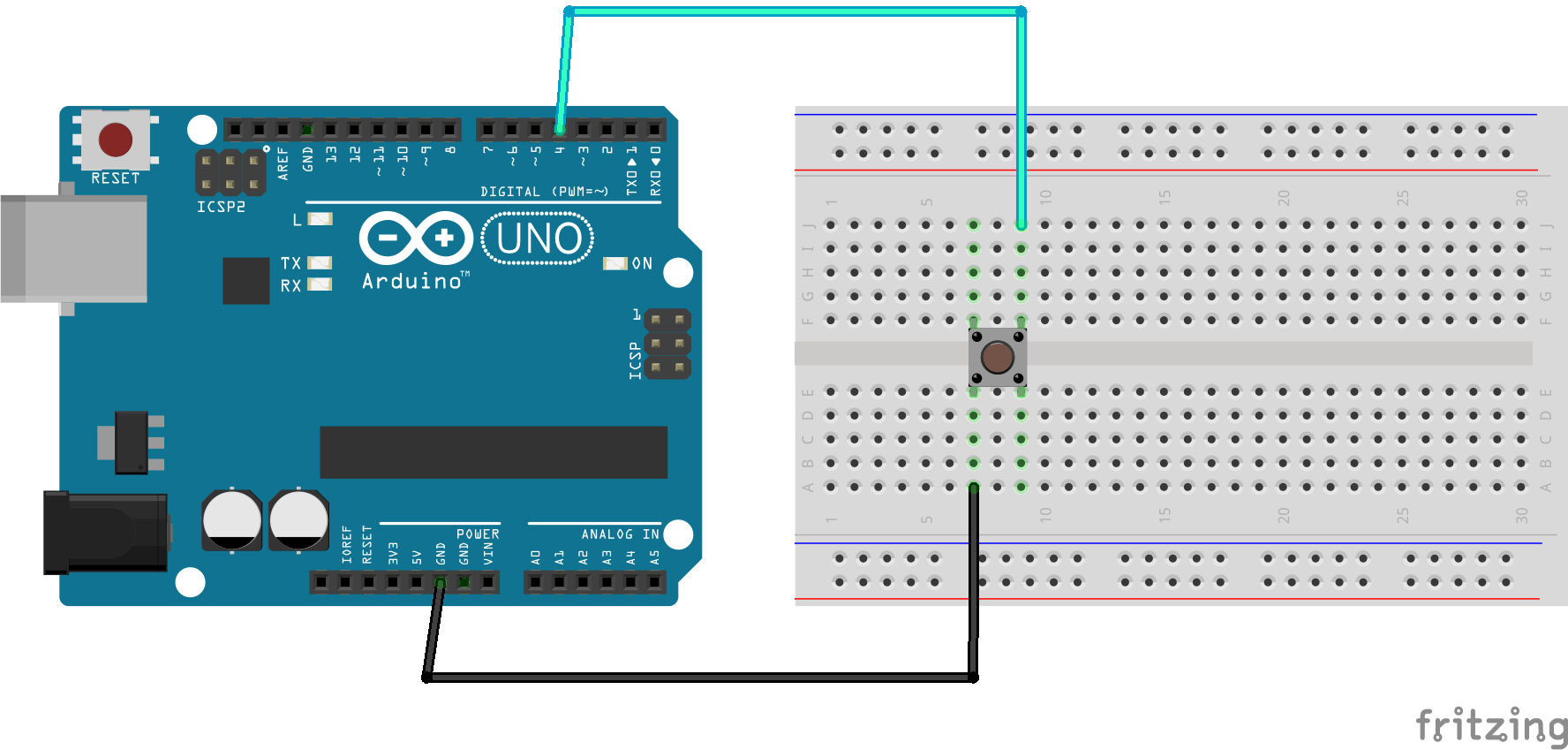
In this lesson you'll learn:
- Direct connection issues
- Pull-Up resistor principle
- Difference between Pull-Up and Pull-Down
- Digital input reading
Important Warnings!
● Avoid short circuits when connecting buttons
● Always use appropriate resistors
● Ensure correct voltage reading
Initial Attempts
1. Direct Connection
Explanation: The button is directly connected to the Arduino input without using a pull-up or pull-down resistor.
Problem: When the button is not pressed, the input pin is left in a "floating" state. This means it might randomly pick up noise, causing **unpredictable behavior**.
Solution: Use a **pull-up resistor** to ensure the input is always in a defined state when the button is not pressed.
2. Dual Connection
Explanation: The button is wired so that it connects both VCC and GND simultaneously.
Problem: When the button is pressed, it directly **shorts VCC to GND**, causing a short circuit that can damage components.
Solution: Use a proper circuit with a pull-up or pull-down resistor to prevent short circuits.
Optimal Solution
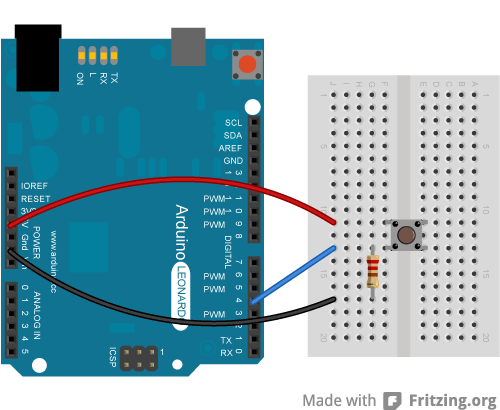
Pull-Up vs Pull-Down Resistors
Type | Pull-Up | Pull-Down |
---|---|---|
Default State | HIGH | LOW |
When Pressed | LOW | HIGH |
Resistor Position | Between VCC and button | Between GND and button |
Programming Examples
// External Pull-Up resistor reading
const int buttonPin = 2;
void setup() {
Serial.begin(9600);
pinMode(buttonPin, INPUT);
}
void loop() {
int state = digitalRead(buttonPin);
if(state == HIGH) {
Serial.println("Button pressed");
} else {
Serial.println("Button released");
}
delay(100);
}
// Using internal Pull-Up resistor
const int buttonPin = 2;
void setup() {
Serial.begin(9600);
pinMode(buttonPin, INPUT_PULLUP);
}
void loop() {
int state = digitalRead(buttonPin);
// State is inverted when using INPUT_PULLUP
if(state == LOW) {
Serial.println("Button pressed");
} else {
Serial.println("Button released");
}
delay(100);
}
Resistor Value Calculation
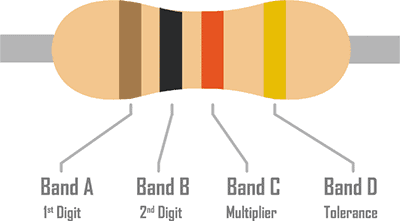
Select colors based on the bands:
First Band - First Digit:
Second Band - Second Digit:
Multiplier:
Tolerance: